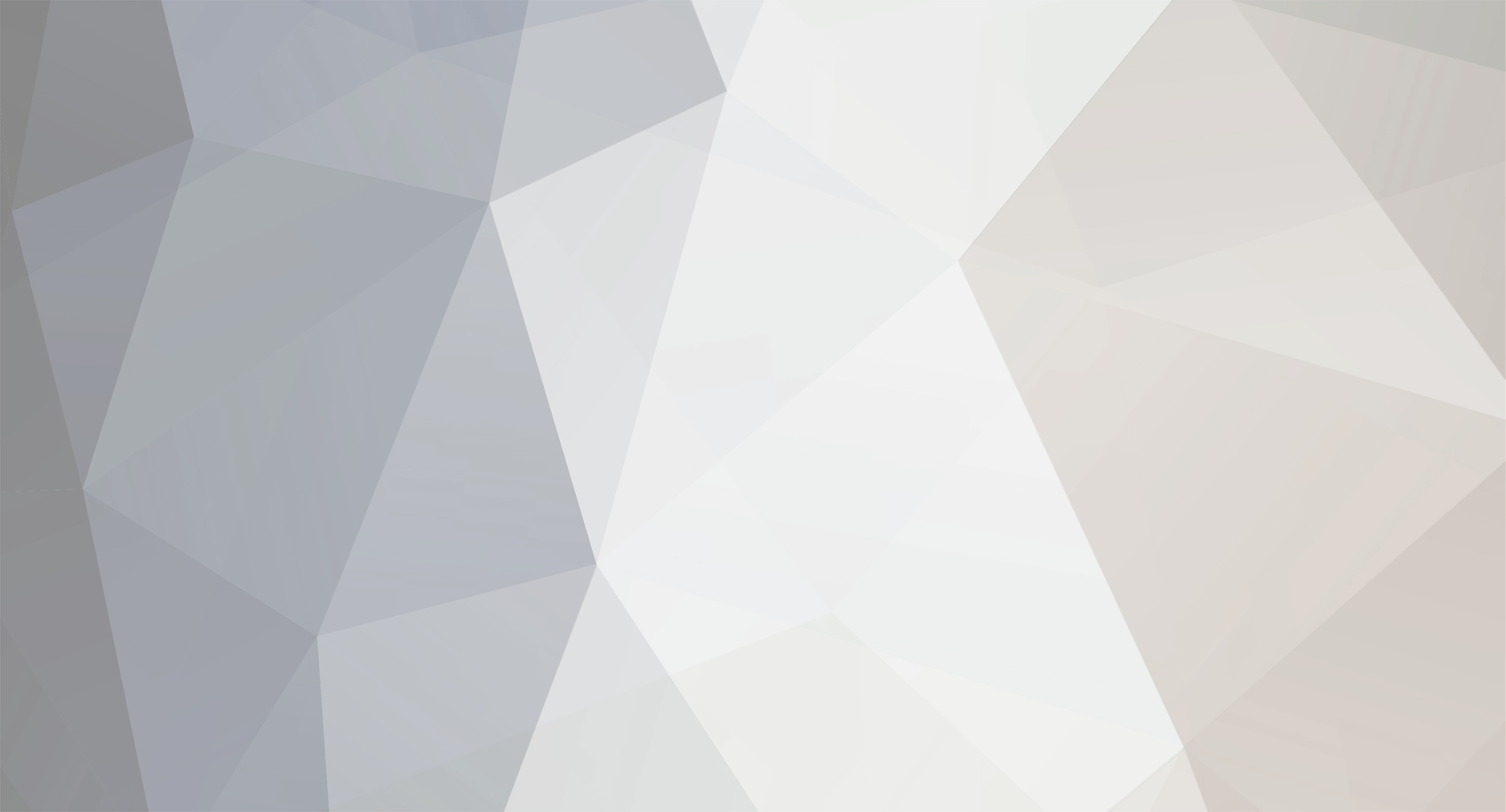
Tekk
Active Members-
Posts
78 -
Joined
Recent Profile Visitors
432 profile views
Tekk's Achievements
-
spudw2k reacted to a post in a topic: Event when window becomes inactive
-
Event when window becomes inactive
Tekk replied to spudw2k's topic in AutoIt General Help and Support
https://docs.microsoft.com/en-us/windows/win32/inputdev/wm-activate -
emendelson reacted to a post in a topic: Folder watcher doesn't work when folder has more than a few files
-
I had some time and threw together a non-blocking version of ReadDirectoryChangesW. More watch flags can be added if you need other notifications (see here). I did hardly any error checking, I'll leave that up to you if you decide to use this method. #include <WinAPI.au3> Global Const $WATCH_PATH = "D:\Desktop\Test" Global $t_Overlapped, $g_hDirectory, $g_hCompletion, $g_pCompletion, $g_pDataBuffer Global Const $BUFFER_LENGTH = 4096 Global Const $NOTIFY_FLAGS = BitOR($FILE_NOTIFY_CHANGE_FILE_NAME, $FILE_NOTIFY_CHANGE_DIR_NAME) _EntryPoint() Func _EntryPoint() $t_Overlapped = DllStructCreate($tagOVERLAPPED) $g_hDirectory = _WinAPI_CreateFileEx($WATCH_PATH, $OPEN_EXISTING, $FILE_LIST_DIRECTORY, _ BitOR($FILE_SHARE_READ, $FILE_SHARE_WRITE, $FILE_SHARE_DELETE), _ BitOR($FILE_FLAG_BACKUP_SEMANTICS, $FILE_FLAG_OVERLAPPED)) $g_hCompletion = DllCallbackRegister(_CompletionRoutine, "NONE", "DWORD;DWORD;PTR") $g_pCompletion = DllCallbackGetPtr($g_hCompletion) $g_pDataBuffer = _WinAPI_CreateBuffer($BUFFER_LENGTH) _ReadDirectoryChanges($g_hDirectory, $NOTIFY_FLAGS, $g_pDataBuffer, $BUFFER_LENGTH, 0, $t_Overlapped, $g_pCompletion) OnAutoItExitRegister(_FreeResources) While 1 _SleepEx(10, 1) ; Required! Thread must enter an alertable state. You could call this in an adlib if you dont require a loop. ; do loop stuff here WEnd EndFunc ;~ Make decisions on file changes in here Func _FileNotifyUser($nFileAction, $sFileName) Switch $nFileAction Case $FILE_ACTION_ADDED ConsoleWrite("File added:" & $sFileName & @CRLF) Case $FILE_ACTION_REMOVED ConsoleWrite("File removed:" & $sFileName & @CRLF) Case $FILE_ACTION_RENAMED_OLD_NAME ConsoleWrite("File renamed from:" & $sFileName & @CRLF) Case $FILE_ACTION_RENAMED_NEW_NAME ConsoleWrite("File renamed to:" & $sFileName & @CRLF) EndSwitch EndFunc Func _FreeResources() _CancelIoEx($g_hDirectory, $t_Overlapped) _WinAPI_CloseHandle($g_hDirectory) _WinAPI_FreeMemory($g_pDataBuffer) DllCallbackFree($g_hCompletion) $t_Overlapped = 0 EndFunc #region internal Func _CompletionRoutine($nError, $nTransfered, $pOverlapped) Local $pBuffer = $g_pDataBuffer Local $tNotify Local $nLength While 1 $nLength = DllStructGetData(DllStructCreate("DWORD", $pBuffer + 8), 1) $tNotify = DllStructCreate("DWORD NextOffset;DWORD Action;DWORD NameLength;WCHAR FileName[" & $nLength / 2 & "]", $pBuffer) _FileNotifyUser($tNotify.Action, $tNotify.FileName) If $tNotify.NextOffset = 0 Then ExitLoop Else $pBuffer += $tNotify.NextOffset EndIf WEnd _ReadDirectoryChanges($g_hDirectory, $NOTIFY_FLAGS, $g_pDataBuffer, $BUFFER_LENGTH, 0, $t_Overlapped, $g_pCompletion) EndFunc Func _ReadDirectoryChanges($hDirectory, $iFilter, $pBuffer, $iLength, $bSubtree = 0, $tOverlapped = 0, $pCompletion = 0) Local $aRet = DllCall('kernel32.dll', 'bool', 'ReadDirectoryChangesW', 'handle', $hDirectory, 'struct*', $pBuffer, _ 'dword', $iLength - Mod($iLength, 4), 'bool', $bSubtree, 'dword', $iFilter, 'dword*', 0, 'struct*', $tOverlapped, 'PTR', $pCompletion) If @error Or Not $aRet[0] Then Return SetError(@error + 10, @extended, 0) Return SetExtended(_WinAPI_GetLastError(), $aRet[0]) EndFunc Func _SleepEx($nMilliseconds, $bAlertable = 0) Local $aRet = DllCall('kernel32.dll', 'dword', 'SleepEx', 'dword', $nMilliseconds, 'bool', $bAlertable) If @error Then Return SetError(@error, @extended, 0) Return $aRet[0] EndFunc Func _CancelIoEx($hFile, $tOverlapped) Local $aRet = DllCall('kernel32.dll', 'bool', 'CancelIoEx', 'handle', $hFile, 'struct*', $tOverlapped) If @error Then Return SetError(@error, @extended, 0) Return $aRet[0] EndFunc #endregion internal
-
It should be more like .. Global $g_aResult = DllCall("kernel32", "BOOL", "Wow64DisableWow64FsRedirection", "PTR*", 0) If True = $g_aResult[0] Then ;~ do file stuff here. DllCall("kernel32", "BOOLEAN", "Wow64RevertWow64FsRedirection", "BOOLEAN", $g_aResult[1]) EndIf Wow64DisableWow64FsRedirection fails for me when I try to call it with an invalid pointer value. You can see an example of its use here. #include <WinAPIFiles.au3> _WinAPI_Wow64EnableWow64FsRedirection ( $bEnable ) The above is a wrapper for Wow64EnableWow64FsRedirection which is a much more simple approach. However documentation states "This function may not work reliably when there are nested calls." I just wanted to bring attention to your posted code failing, at least on my system. I don't mean to derail the thread.
-
@Subz BOOL Wow64DisableWow64FsRedirection( PVOID *OldValue ); I assume you intended to "DllCall" Wow64EnableWow64FsRedirection as your help link points to it. Your posted "DllCall" is very wrong.
-
Another possible solution. #AutoIt3Wrapper_Change2CUI=y ShellExecute("notepad.exe") Global $g_cbHandler = DllCallbackRegister(_HandlerRoutine, "BOOL", "DWORD") ;~ https://docs.microsoft.com/en-us/windows/console/setconsolectrlhandler DllCall("kernel32", "BOOL", "SetConsoleCtrlHandler", "PTR", DllCallbackGetPtr($g_cbHandler), "BOOL", True) ProcessWaitClose(@AutoItPID) Func _HandlerRoutine($dwCtrlType) ProcessClose("notepad.exe") EndFunc
-
wolflake reacted to a post in a topic: _Timer_SetTimer question Solved
-
The problem is likely due to "_Timer_KillTimer" being called while inside the callback. Inside "_Timer_KillTimer" is a call to "DllCallbackFree" which is effectively freeing memory which is due to be executed when called inside the callback. I would recommend using "AdlibRegister" and "AdlibUnRegister" which are really just built in versions of the same thing. I've not tested it but at face value I do believe that it will solve your problem. Edit: As a side note you might reconsider your program flow. All callback type functions should be returned from as quickly as possible. Hanging in them, which your posted code does, can lead to unexpected issues.
-
I made the assumption that the file was not being created at all. Your question clearly states that it is. Perhaps I should work on my reading comprehension, lol. Anyways, I believe your problem is being caused by FILE_FLAG_DELETE_ON_CLOSE. Microsoft states: "If there are existing open handles to a file, the call fails unless they were all opened with the FILE_SHARE_DELETE share mode". Perhaps the file is not locked, just prevented from being opened without the proper flags?
-
The problem could be that you are trying to create the file at the root of C:\\. Doing so requires elevation.
-
Earthshine reacted to a post in a topic: RdRand - calling Intel's on-chip RNG
-
Right, but it was the check for support that I was questioning. Sorry for being unclear.
-
I understand, I was only pointing it out. As for the title clearly stating this, I would argue that AMD chips, at least the chips in question here, execute the Intel x86 instruction set. AMD's Zen and newer processors will support this instruction. The developer should have simply checked for the feature bit to be set and disregarded manufacturer. I don't mean to argue, I just wanted to point out that it fails to work in a case where it should work. I thank you for wrapping the library for use in AutoIt.
-
Just wanted to point out that "rdrand_supported()" will return false when executed on AMD Zen processors even though rdrand is a supported instruction. The dll author did not plan for the future.
-
Is there a proper way to use %ProgramFiles%?
Tekk replied to qwert's topic in AutoIt GUI Help and Support
You CAN do this with "Opt" or "AutoItSetOption". Opt("ExpandEnvStrings", 1) MsgBox(0, "ExpandEnvStrings", "%ProgramFiles%\Windows NT\Accessories\wordpad.exe") -
how to DllCall() for a function that returns a whole struct?
Tekk replied to Yorgo's topic in AutoIt General Help and Support
A function cannot return a structure, not with conventional calling conventions, however they may return them by reference. It's just not how x86 CPU's are designed work at low level, most return values are given back in CPU registers where a structure generally will not fit. Just because the source code looks as if it does, that is not what the compiler is really doing. ftdi_get_library_version accepts one parameter; a pointer to a ftdi_version_info structure. It also uses cdecl calling convention. I know this because I loaded the library into a debugger. The following example does work, I have tested it. I chose to load the structure into an array for ease of display. #include <Array.au3> Global $g_hDll = DllOpen("libftdi1.dll") If $g_hDll < 0 Then MsgBox(0x40000, "Error!", "Failed to load library!") Else Global $g_aVersion = ftdi_get_library_version() _ArrayDisplay($g_aVersion) EndIf Func ftdi_get_library_version() Local $ftdi_version_info = DllStructCreate("INT major;INT minor;INT micro;PTR version_str;PTR snapshot_str") DllCall($g_hDll, "NONE:cdecl", "ftdi_get_library_version", "STRUCT*", $ftdi_version_info) Local $aReturn[5][2] = [["major"], ["minor"], ["micro"], ["version_str"], ["snapshot_str"]] $aReturn[0][1] = $ftdi_version_info.major $aReturn[1][1] = $ftdi_version_info.minor $aReturn[2][1] = $ftdi_version_info.micro $aReturn[3][1] = DllStructGetData(DllStructCreate("CHAR[255]", $ftdi_version_info.version_str ), 1) $aReturn[4][1] = DllStructGetData(DllStructCreate("CHAR[255]", $ftdi_version_info.snapshot_str), 1) Return $aReturn EndFunc -
_WinAPI_FormatMessage() - useless line of code?
Tekk replied to jrsofsd's topic in AutoIt General Help and Support
Looks used to me, notice that $pBuffer is a ref. -
Using a string literally for function parameters
Tekk replied to IAMK's topic in AutoIt General Help and Support
$stuff2 = 'Words, 0, 0' _Call(ToolTip, $stuff2) Sleep(2000) Func _Call($fuFunc, $sArgs) Return Call($fuFunc, StringSplit("CallArgArray," & $sArgs, ",", 2)) EndFunc