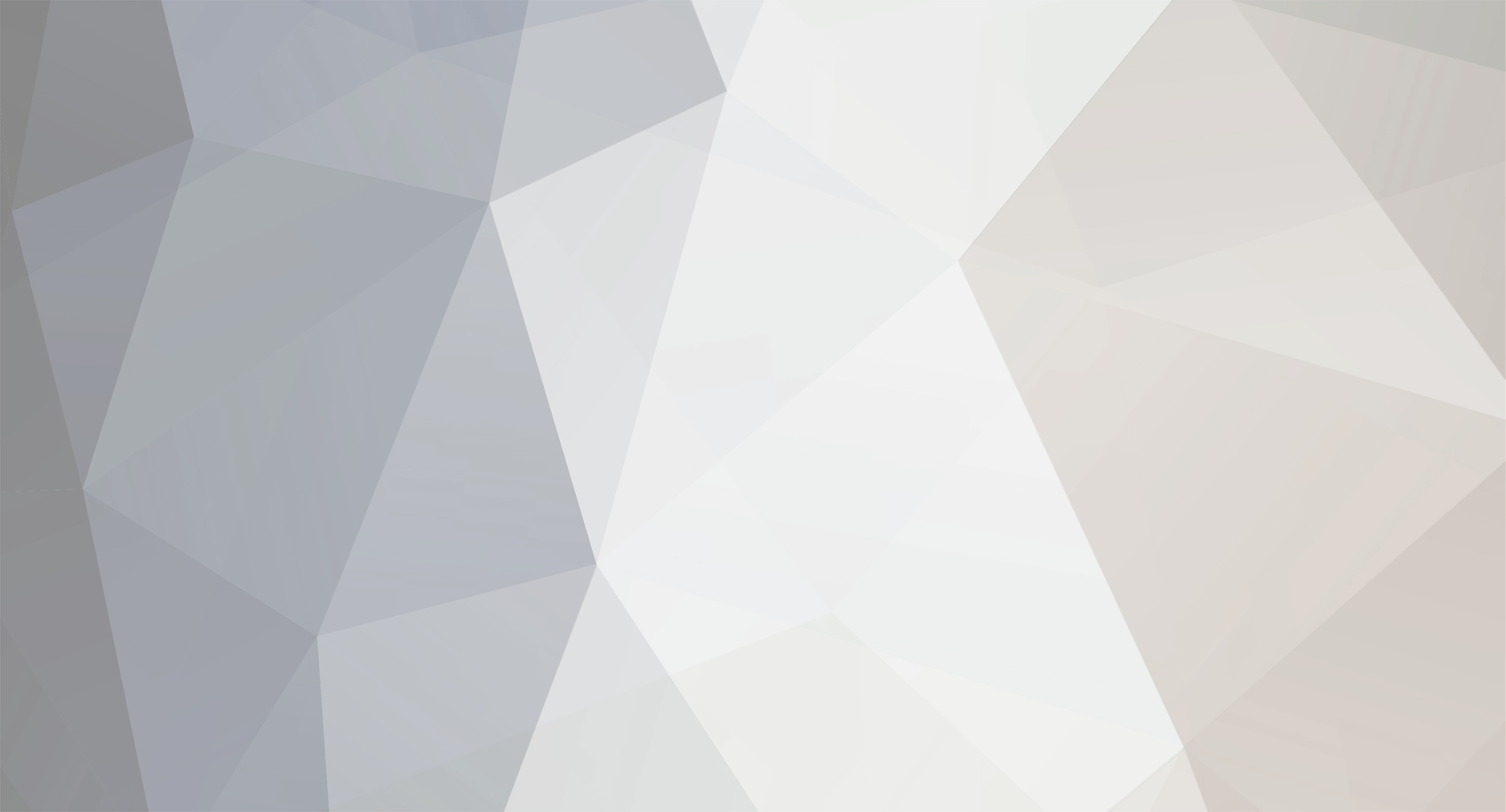
Simucal
Active Members-
Posts
768 -
Joined
-
Last visited
Everything posted by Simucal
-
Minimize application into Task Tray
Simucal replied to Flo's topic in AutoIt General Help and Support
Search for "Tray" in the AutoIt Help file. You'll find all the methods you need. -
Basic Array Management Issues
Simucal replied to programit's topic in AutoIt General Help and Support
If you insist on using the 2d array I guess you could do something like this: #include <Array.au3> ; $PackedItems[Item][Quantity] Global $PackedItems[3][2] $PackedItems[0][0] = "Basketball^12" $PackedItems[0][1] = 12 $PackedItems[1][0] = "Baseball&@##!" $PackedItems[1][1] = 22 $PackedItems[2][0] = "Football^12112" $PackedItems[2][1] = 43 While 1 $sItemName = InputBox("Enter Item", "Enter an item name to search for!") ; Do a partial search on PackedItems array $iSearchIndex = _ArraySearch($PackedItems, $sItemName, 0, UBound($PackedItems), 0, 1) If $iSearchIndex = -1 Then MsgBox(0, "Not Found", $sItemName & " was not found in $PackedItems. Adding it.") $iItemQuantity = InputBox("Enter Quantity", "Enter an item quantity") ReDim $PackedItems[UBound($PackedItems) + 1][2] $PackedItems[UBound($PackedItems) - 1][0] = $sItemName $PackedItems[UBound($PackedItems) - 1][1] = $iItemQuantity MsgBox(0, "Item added", "Item: " & $sItemName & " Qty: " & $iItemQuantity & " has been added!") Else MsgBox(0, "Found!", $sItemName & " was found in $PackedItems at [" & $iSearchIndex & "][0]"&@CRLF&"Item Name:"&$PackedItems[$iSearchIndex][0]&@CRLF&"Quantity:" &$PackedItems[$iSearchIndex][1]) EndIf WEnd Try searching for "Basketball", you'll get a hit on "Basketball^12". If it doesn't get a hit on what you search it will ask you for a quantity, redim the array and add it. It should be noted if you have a large number of elements redimm'ing the array is a computationally expensive process so it could be slow. Similarly, your searching might be slow and you could speed it up considerably using a Binary Search if you sorted your data. As far as your search not returning "Basketball^12" when you search for "Basketball", you need to use a Partial search. -
I'd say it's possible but I don't honestly think you are going to do it. AutoIt definately isn't the best enviornment for something like that.. you should probably focus your attention elsewhere. There are several types of aimbots: Graphics Driver Aimbots (DirectX/OpenGL)Memory/Client AimbotsColor AimbotsPacket Sniffing Bots (defunct/not really around any longer)With the Graphics Driver Aimbots you hijack control of the rendering library, find out the x, y, z location of a player and perform some matrices caclulations to convert that into a two dimensional point on your screen. These are largely detectable by modern, online games. With Memory/Client Reading Aimbots, it reads your viewing/camera angle and the x/y/z positions of players around you. Then you bust out some trigonometry to calculate the angle at which you should be pointing your weapon, taking into consideration things like lead time/latency, hitboxes, etc. It isn't a trivial thing.
-
Artificial Intelligence - Bot path finding
Simucal replied to Toady's topic in AutoIt Example Scripts
Toady, Very nice application! It seems like a very good learning tool for those new to Graphs, Graph Traversal, and Pathing Algorithms. If you feel like expanding it you might consider having a few other algorithms that the user can select and run against your maze so the user can see the physical difference of the nodes that each one evaluates. Maybe something like: * Dijkstra's Algorithm (Really A* is a specialized variant of Dijkastra's) * B-Star (B*) - Storing Intervals of nodes of the tree * D-Star (D*) - As far as I know places like Garmin/TomTom use specialized D* for finding best paths in partially known enviornments to plan your driving route. * Depth and Breadth first traversal. Along with visually showing the user the nodes it is considering along the way. and apart from path finding.. implementing something like the Floyd-Warshall algorithm to find the shortest point between all vertices would be kind of cool. Might be slow at O(V^3).. but with this small a graph it shouldn't be too bad. Another expansion you could do is to find a flash game that is maze like and embed it into an autoit app. You can then have a maze-solver utilizing Depth-First or one of your Best-First algorithms. Watching it automate and solve the flash-games mazes would definately be cool! -
[resolved] string regex
Simucal replied to anystupidassname's topic in AutoIt General Help and Support
#include <Array.au3> $sText = "asldkjfsadf1234567890101213dsflkjsdfsdf2!" $aResult = StringRegExp($sText, "\d{12,16}", 1) If IsArray($aResult) Then _ArrayDisplay($aResult) Else MsgBox(0, "Error", "No 12-16 digits found!") EndIf Obviously you'll need to use ClipGet to get the clipboard text. -
Func TOP10() _GUICtrlListBox_ResetContent($LISTBOX1) $SOURCE = _INETGETSOURCE("http://www.swedvdr.org/browse.php?sort=7&type=desc") $Top10 = _StringBetween($SOURCE,"'><b>","</b></font></a><br>") _GUICtrlListBox_AddString($LISTBOX1, "________TOP10_______") For $I = 1 To UBound($Top10) - 1 _GUICtrlListBox_AddString($LISTBOX1, $Top10 [$I]) Next EndFunc Something like that. You weren't too far off. EDIT: My bad, was beaten to it
-
[Solved] TCP and Multiple Sockets
Simucal replied to Simucal's topic in AutoIt General Help and Support
Solved this myself. I had no TCPStartup() in my client, stupid mistake. Sorry for the post. -
Alright, I've written two small, really basic scripts for server/client that will allow up to 100 concurrent sockets/connections. I've searched, I've read the threads.. I don't see what I'm doing wrong. I'm TCPAccepting all connections to an array, etc, etc. However, I'm never able to connect to the server. What mistake am I making? Every time the connection I attempt to make to my server will immediately return -1. Anyway, here are the scripts: Client: #include <GuiConstantsEx.au3> $hClient = GUICreate("TCP Client", 387, 105, 260, 281) $oSend = GUICtrlCreateButton("Send", 256, 64, 89, 25, 0) $oInput = GUICtrlCreateInput("", 32, 64, 217, 21) $oConnect = GUICtrlCreateButton("Connect", 32, 16, 105, 33, 0) $oDisconnect = GUICtrlCreateButton("Disconnect", 144, 16, 105, 33, 0) GUISetState(@SW_SHOW, $hClient) Global $oSocket = -1 While 1 $nMsg = GUIGetMsg() Switch $nMsg Case $GUI_EVENT_CLOSE Exit Case $oConnect _OpenSocket() Case $oDisconnect _CloseSocket() Case $oSend _SendData() Case $oInput _SendData() EndSwitch WEnd Func _SendData() If $oSocket <> -1 Then TCPSend($oSocket, GUICtrlRead($oInput)) Else MsgBox(0, "Error", "You must be connected first!") EndIf EndFunc ;==>_SendData Func _CloseSocket() If $oSocket <> -1 Then TCPCloseSocket($oSocket) Else MsgBox(0, "Error", "Socket is already disconnected!") EndIf EndFunc ;==>_CloseSocket Func _OpenSocket() If $oSocket <> -1 Then MsgBox(0, "Error", "You are already connected!") Else $oSocket = TCPConnect("127.0.0.1", "2001") If $oSocket = -1 Then MsgBox(0, "Error", "Can not connect to server!"&@CR&"@Error = "&@Error) Else TCPSend($oSocket, "You are connected to the server!") EndIf EndIf EndFunc ;==>_OpenSocketoÝ÷ Ø ÝIêïz¶®¶sdvÆö&Âb33c¶7W'&VçE6ö6¶WBÒÂb33c¶ôÆ7FVå6ö6¶WBÂb33c¶ô6öææV7FVE6ö6¶WE³Ð ¥õD57F'E6W'fW" ¥vÆR b33c¶ô6öææV7FVE6ö6¶WE²b33c¶7W'&VçE6ö6¶WEÒÒD566WBb33c¶ôÆ7FVå6ö6¶WB 6öç6öÆUw&FRb33c¶ô6öææV7FVE6ö6¶WE²b33c¶7W'&VçE6ö6¶WEÒf×´5" bb33c¶ô6öææV7FVE6ö6¶WE²b33c¶7W'&VçE6ö6¶WEÒfÇC²fwC²ÓFVà ×6t&÷ÂgV÷CµD5gV÷C²ÂgV÷C´6ÆVçB6öææV7FVBöâ6ö6¶WB2gV÷C²fײb33c¶7W'&VçE6ö6¶WB b33c¶7W'&VçE6ö6¶WBÒb33c¶7W'&VçE6ö6¶WB² VæD` f÷"b33c¶ÒFò bb33c¶ô6öææV7FVE6ö6¶WE²b33c¶ÒfÇC²fwC²Ó÷"b33c¶ô6öææV7FVE6ö6¶WE²b33c¶ÒfÇC²fwC²gV÷C²gV÷C²FVà b33c·5&W7bÒD5&V7bb33c¶ô6öææV7FVE6ö6¶WE²b33c¶ÒÂS bb33c·5&W7bfÇC²fwC²gV÷C²gV÷C²FVâ×6t&÷ÂgV÷CµD5gV÷C²ÂgV÷C´FF&V6VfVBg&öÒ6ö6¶WB3¢gV÷C²fײb33c¶fײ5"fײgV÷C´FF¢gV÷C²fײb33c·5&W7b VæD` æW@ 6ÆVW#¥tVæ@ ¤gVæ2õD57F'E6W'fW" Æö6Âb33c·5&W7VÇ@ b33c·5&W7VÇBÒD57F'GW bb33c·5&W7VÇBÒFVà ×6t&÷ÂgV÷C´W'&÷"gV÷C²ÂgV÷CµVæ&ÆRFò7F'GWD56W'f6W2b333²gV÷C² W@ VæD` b33c¶ôÆ7FVå6ö6¶WBÒD5Æ7FVâgV÷C³#rãããgV÷C²ÂgV÷C³#gV÷C²ÂgV÷C³gV÷C² bb33c¶ôÆ7FVå6ö6¶WBÒÓFVà ×6t&÷ÂgV÷C´W'&÷"gV÷C²ÂgV÷CµVæ&ÆRFò7F'BÆ7FVææröâ÷'B#gV÷C² W@ VæD`¤VæDgVæ2³ÓÒfwCµõD57F'E6W'fW This is more of a proof of concept for myself so if I can keep the structure as close to what it is now it would be better. Thank you and I look forward to hearing any comments people have.
-
Yea
-
Search string from starting position (parsing)
Simucal replied to dogsurfer's topic in AutoIt General Help and Support
I would just use StringInStr to find your "x", then trim left of that and StringInString for "y". Although you could probably use a regex to simplify this process with a single line of code. -
Here it is an a UDF format in case anyone ever needs this: ; #_GetSid# ;=============================================================================== ; ; Name...........: _GetSid ; Description....: Returns a specified users SID (if none specified, will return current users Sid) ; Syntax.........: _GetSid([$sUsername]) ; Parameters.....: [Optional] $sUsername - The username of the sid you wish to retrieve ; Return values..: Success - Returns Current/Specified users SID ; Failure - Returns 0 and Sets @Error: ; |0 - No error. ; |1 - Specified username not found on system. ; |2 - User running this function does not have permission to access WMIService ; Author.........: Simucal (Matthew McDole) ; Modified.......: 02.09.2008 ; Remarks........: ; Related........: ; Link...........: ; Example........: ; ;========================================================================================== Func _GetSid($sUsername = @UserName) Local $oWMIService Local $oColAccounts $oWMIService = ObjGet("winmgmts:\\.\root\cimv2") If Not IsObj($oWMIService) Then SetError(2) Return 0 EndIf $oColAccounts = $oWMIService.ExecQuery("Select * From Win32_UserAccount") For $oAccount In $oColAccounts If $oAccount.Name = $sUsername Then SetError(0) Return $oAccount.Sid EndIf Next SetError(1) Return 0 EndFunc ;==>_GetSidoÝ÷ ØLZ^jëh×6ConsoleWrite(_GetSid()&@CR) ; Display current users sid ConsoleWrite(_GetSid("HelpAssistant")&@CR) ; Display specified users sid And it would work fine.
-
Global $oAccount, $sComputer = "." $oWMIService = ObjGet("winmgmts:\\"&$sComputer&"\root\cimv2") $oColAccounts = $oWMIService.ExecQuery("Select * From Win32_UserAccount") For $oAccount In $oColAccounts ConsoleWrite("Username: " & $oAccount.Name & @CR) ConsoleWrite("SID: " & $oAccount.SID & @CR&@CR) Next EDIT: Made small change so it outputs all users.
-
I'm not trying to gather any personal (usuable at least) information from the user whatsoever. I also plan on telling them that my program reports anonymous usage information to me. They can then decide if they still want to use the script or not. My program has 660 regisistered users atm, but I'm really curious about how large the number of total users is when I take into account the people who never bothered to register/post. I was more looking for any other considerations into my approach (technical) rather than moralistic warnings about wether or not to do it. I've already decided to do it. One thing I considered was generating a unique hardware id for each user and encrypting that hardware id and sending me some sort of unique,encrypted,hash. Then I can keep a database of unique hash's and that will give me a rough estimate of the unique computers my script is installed on. Any other thoughts/ideas?
-
Ok... anyone else?
-
It is always in the best interest of the community for you to post whatever solutions you find... in the event someone looks this thread up down the line.
-
I have a program/script currently released and I'm interested in adding a function in it that will report to me how many people at any given time are using it. I was curious at how some of you might implement this. Do you think it (my script) should TCPConnect to a server I have setup here every x minutes and send some particular information.. and if my server doesn't receieve any info from any given person for x minutes then it will drop from the list? Ideally, I would like to be able to pull up a list and see who is using my program at any given time. I'm not asking for any code, just some thoughts about implementation. What would you guys consider the best method to do something like this? Thanks, Sim
-
Best way to hide/show external window?
Simucal replied to qwert's topic in AutoIt General Help and Support
What program is it? -
Best way to hide/show external window?
Simucal replied to qwert's topic in AutoIt General Help and Support
In case you are interested.. here is a script I wrote a couple days ago that lists windows of a particular window title into a Listview, shows their hWnd, Instance, and visibility state.. and has a button to change their visibility state. #Include <GUIConstants.au3> #Include <GuiListView.au3> $hWindowList = GUICreate("Hide/Show", 265, 140, 596, 604) $oWindowList = GUICtrlCreateListView("Instance|hWnd|State", 8, 8, 249, 89) GUICtrlSendMsg(-1, 0x101E, 0, 100) GUICtrlSendMsg(-1, 0x101E, 1, 70) GUICtrlSendMsg(-1, 0x101E, 2, 70) $oHideShow = GUICtrlCreateButton("Hide/Show", 8, 104, 113, 25, 0) $oHideShowCancel = GUICtrlCreateButton("Cancel", 128, 104, 113, 25, 0) GUISetState(@SW_SHOW, $hWindowList) Global $aOldWinList, $aNewWinList While 1 $nMsg = GUIGetMsg() Switch $nMsg Case $GUI_EVENT_CLOSE Exit Case $oHideShow _HideShow() EndSwitch _PopulateWindowList() Sleep(20) WEnd Func _HideShow() Local $iSelectedIndex Local $aTemp ConsoleWrite("Selected: " & _GuiCtrlListView_GetNextItem($oWindowList, -1, 0, 8) & @CR) $iSelectedIndex = _GuiCtrlListView_GetNextItem($oWindowList, -1, 0, 8) If $iSelectedIndex <> -1 Then $aTemp = StringSplit(_GuiCtrlListView_GetItemTextString($oWindowList, $iSelectedIndex), "|") If IsArray($aTemp) And $aTemp[0]=3 Then If $aTemp[3] = "Visible" Then ConsoleWrite("WinSetState("&$aTemp[2]&")"&@CR) WinSetState(HWnd($aTemp[2]), "", @SW_HIDE) Else WinSetState(HWnd($aTemp[2]), "", @SW_SHOW) EndIf EndIf Else MsgBox(0, "Error", "You must select a window to hide/show!") EndIf EndFunc ;==>_HideShow Func _PopulateWindowList() Local $aWinList $aWinList = WinList("Untitled - Notepad") If IsArray($aWinList) And UBound($aWinList) > 1 Then ; Convert the current window list to my array format [hwnd][visible/hidden] Dim $aNewWinList[UBound($aWinList, 1)][2] For $i = 1 To UBound($aWinList) - 1 $aNewWinList[$i][0] = $aWinList[$i][1] $aNewWinList[$i][1] = _ReturnVisibility($aWinList[$i][1]) Next If Not _Array2DimCompare($aNewWinList, $aOldWinList) Then ;Compare new window list to the old window list previously applied to the listview If _GUICtrlListView_GetItemCount($oWindowList) > 0 Then _GUICtrlListView_DeleteAllItems(GUICtrlGetHandle($oWindowList)) ; Clear the listview if it isnt already For $i = 1 To UBound($aWinList, 1) - 1 ; Fill the listview with new updated window list/states GUICtrlCreateListViewItem("Notepad #" & $i & "|" & $aWinList[$i][1] & "|" & _ReturnVisibility($aWinList[$i][1]), $oWindowList) Next $aOldWinList = $aNewWinList ; Make the new list now the old one. EndIf Else If _GUICtrlListView_GetItemCount($oWindowList) > 0 Then _GUICtrlListView_DeleteAllItems(GUICtrlGetHandle($oWindowList)) ; Clear the listview if it is full and we found no windows EndIf EndFunc ;==>_PopulateWindowList Func _ReturnVisibility($hWND) If WinExists($hWND) Then If BitAND(WinGetState($hWND), 2) Then Return "Visible" Else Return "Hidden" EndIf EndIf EndFunc ;==>_ReturnVisibility Func _Array2DimCompare($Array1, $Array2, $iBase1 = 1, $iBase2 = 0, $iCase = 1) If UBound($Array1, 1) <> UBound($Array2, 1) Then Return SetError(1, 0, 0) If UBound($Array1, 2) <> UBound($Array2, 2) Then Return SetError(2, 0, 0) For $iCC = $iBase1 To UBound($Array1, 1) - 1 For $xCC = $iBase2 To UBound($Array1, 2) - 1 If $iCase Then If Not ($Array1[$iCC][$xCC] == $Array2[$iCC][$xCC]) Then _ Return SetError(3, 0, 0) Else If Not ($Array1[$iCC][$xCC] = $Array2[$iCC][$xCC]) Then _ Return SetError(3, 0, 0) EndIf Next Next Return 1 EndFunc ;==>_Array2DimCompare EDIT: Open up a few blank notepad instances to see them show up in the list. -
I would suggest making a different child gui for each of your situations.. rather than rebuilding it. I have a script with 5 possible windows and they all work fine open at the same time. While I don't use OnEventMode, I dont see that being an issue for you.
-
I remember reading a dev discussion about this.. and I think their concensus was while they wanted to remove the [0] element holding size thing... they decided it would break too many scripts currently out there so they were going to leave it. That is last I heard.
-
Virus through autoit 123 in scite
Simucal replied to DarkSoul's topic in AutoIt General Help and Support
AutoIt files get flagged as viruses all the time. It is just false positives. -
Thanks for your input zedna. I was thinking of doing the same thing, adding the timer to prevent the constant hammering. Also, that arraycompare function is Smoke_n's... I had it labled as such in the comments but I think "Tidy" stripped it. So, major credit to you smoke.
-
#include <Date.au3> Global $iKillTimerDiff, $iHours, $iMins, $iSecs For $i = 1 To 1000 _TicksToTime($iKillTimerDiff, $iHours, $iMins, $iSecs) ToolTip("Monsters Killed: " & $i & @CR & "Kill Time: " & $iMins & "Min "&$iSecs&"Sec", 0, 0) $oKillTimer = TimerInit() _Find() Send("3") _checkhealth() Send("2") Send("T") Send("T") $iKillTimerDiff = TimerDiff($oKillTimer) Next Func _Find() ; Find code goes here EndFunc Func _CheckHealth() ; CheckHealth goes here EndFunc This is an example of something you could do.
-
In the helpfile look for examples using TimerInit() and TimerDiff() ToolTip("This is"&@CR&"on two lines") Sleep(2000)
-
Really, I just feel like a person reaches a certain level of proficiency in scripting/programming to where they can get most of the programs they write to do what they want.. but I'm always sure there are better ways to do things. I feel like I stagnate since I have no peer review of my code and no one to say, "Hey.. you could simplify this or do this more efficently, etc, etc, etc"