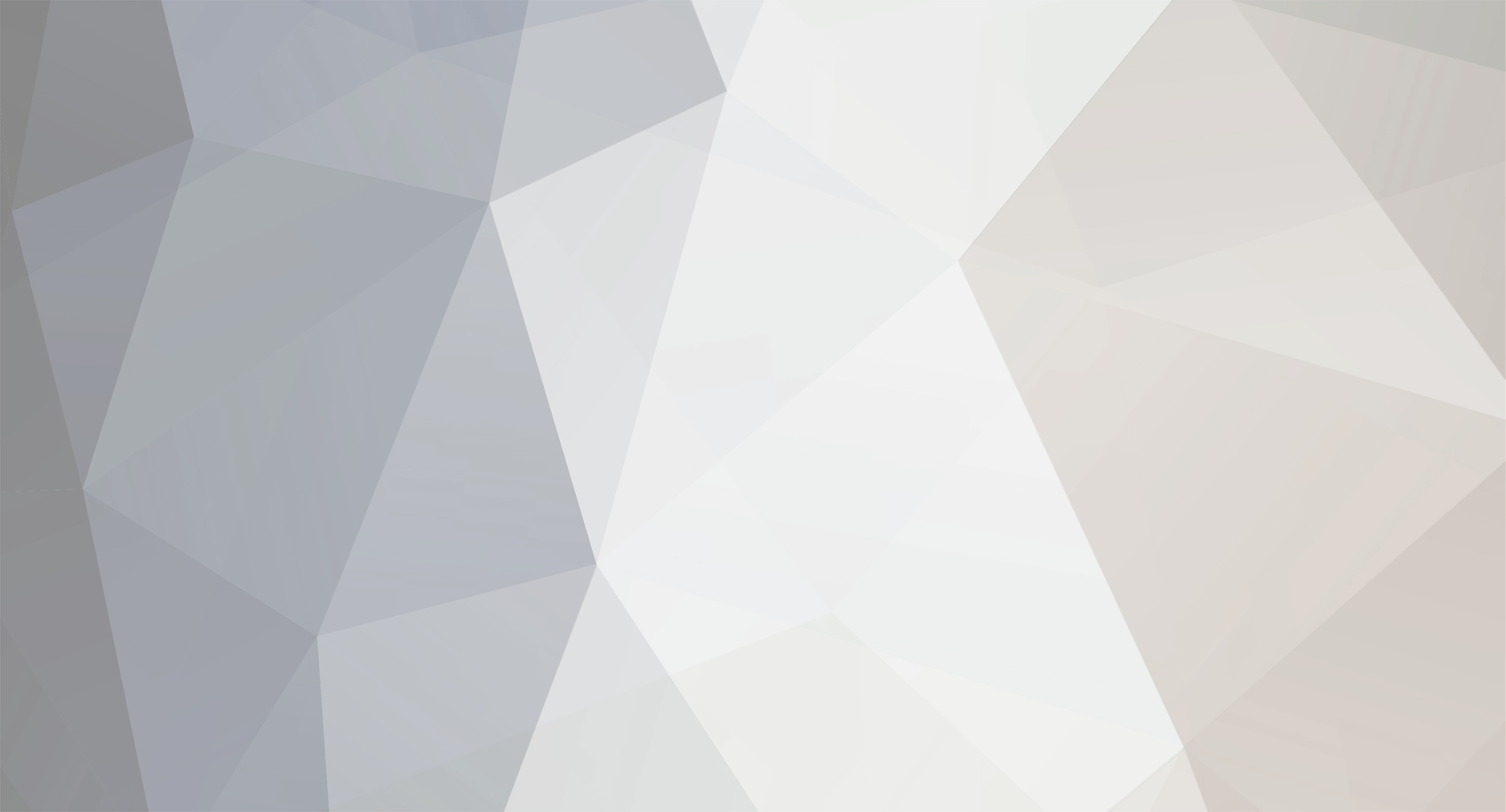
trailwalker
Members-
Posts
17 -
Joined
-
Last visited
Everything posted by trailwalker
-
Sure, this is what I had. Haven't touched it since the original playing around since the GUI issues discouraged me too much and got busy with other things. This would definitely need a lot of cleaning up and more error handling, but this was a working model... I tried to take out everything except the parts that really pertain to the authorization and using it. You can piece it together depending on your application. Func Authorize($verifier_input = "") If $verifier_input = "" Then ; initial auth setup $path = "/oauth/request_token" $postdata = "oauth_consumer_key=" & $oauth_consumer_key $header = 'Authorization: OAuth oauth_consumer_key="' & $oauth_consumer_key & '"' $header &= ',oauth_signature_method="PLAINTEXT"' $header &= ',oauth_timestamp="' & Timestamp() & '"' $header &= ',oauth_nonce="' & Nonce() & '"' $header &= ',oauth_signature="' & $oauth_signature & '"' $response = MakeRequest("POST", $path, $postdata, $header) SetTokenStrings($response[1]) $path = "/oauth/authorize" $postdata = $response[1] $header = "" $link = "https://" & $host & $path & "?" & $postdata ShellExecute($link) Else ; returning setup, process verifier $oauth_verifier = $verifier_input $path = "/oauth/access_token" $postdata = "oauth_consumer_key=" & $oauth_consumer_key $header = 'Authorization: OAuth oauth_consumer_key="' & $oauth_consumer_key & '"' $header &= ',oauth_token="' & $oauth_token & '"' $header &= ',oauth_signature_method="PLAINTEXT"' $header &= ',oauth_timestamp="' & Timestamp() & '"' $header &= ',oauth_nonce="' & Nonce() & '"' $header &= ',oauth_signature="' & $oauth_signature & '"' $header &= ',oauth_verifier="' & $oauth_verifier & '"' $response = MakeRequest("POST", $path, $postdata, $header) SetTokenStrings($response[1]) IniWrite($ini, "auth", "tk", $oauth_token) IniWrite($ini, "auth", "sig", $oauth_signature) AuthStuff("HIDE") Refresh() EndIf EndFunc Func Refresh() ; make sure it isn't too recent first If Timestamp() - $last_refresh > 44 Then $last_refresh = Timestamp() $path = "/api/v1/messages.xml" $postdata = "" $header = 'Authorization: OAuth oauth_consumer_key="' & $oauth_consumer_key & '"' $header &= ',oauth_token="' & $oauth_token & '"' $header &= ',oauth_signature_method="PLAINTEXT"' $header &= ',oauth_timestamp="' & Timestamp() & '"' $header &= ',oauth_nonce="' & Nonce() & '"' $header &= ',oauth_signature="' & $oauth_signature & '"' $response = MakeRequest("GET", $path, $postdata, $header) $last_xml = $response[1] Else MsgBox(0, "", "Too soon to refresh!") EndIf _XMLLoadXML($last_xml) $nNodes = _XMLGetNodeCount("/response/messages/message") For $i = 1 To $nNodes $body = _XMLGetValue("/response/messages/message[" & $i & "]/body/plain") $text = $body[1] Next EndFunc Func Post($s) $path = "/api/v1/messages.xml" $postdata = "body=" & $s ; need to encode this $header = 'Authorization: OAuth oauth_consumer_key="' & $oauth_consumer_key & '"' $header &= ',oauth_token="' & $oauth_token & '"' $header &= ',oauth_signature_method="PLAINTEXT"' $header &= ',oauth_timestamp="' & Timestamp() & '"' $header &= ',oauth_nonce="' & Nonce() & '"' $header &= ',oauth_signature="' & $oauth_signature & '"' $header &= ' Content-Type: application/x-www-form-urlencoded' $response = MakeRequest("POST", $path, $postdata, $header) _ArrayDisplay($response) ;~ Refresh() EndFunc Func Startup() ; see if ini file is there first If Not FileExists($ini) Then FileOpen($ini, 10) FileClose($ini) EndIf $temp_token = IniRead($ini, "auth", "tk", "") $temp_signature = IniRead($ini, "auth", "sig", "") If $temp_token = "" Then ; if blank, do initial authorization first AuthStuff("SHOW") MsgBox(16, "Initial setup required", "It appears that you have not set up authorization.") Authorize() Else $oauth_token = $temp_token $oauth_signature = $temp_signature ;~ Refresh() EndIf EndFunc Func MakeRequest($req_type, $req_path, $req_postdata, $req_header, $req_return_type = 1) ; set up connection, pass arguments and return something ... options for returning? Local $t_or_f Switch $req_return_type Case 1 ; default return type $t_or_f = "True" Case 2 $t_or_f = "False" EndSwitch Local $session = _WinHttpOpen() Local $connection = _WinHttpConnect($session, $host) Local $response = _WinHttpSimpleSSLRequest($connection, $req_type, $req_path, $req_postdata, Default, $req_header, $t_or_f) _WinHttpCloseHandle($connection) _WinHttpCloseHandle($session) Return $response EndFunc Func SetTokenStrings($s) ; we need oauth_token and oauth_token_secret from string and might be out of order $aResponse = StringSplit($s, "&") For $i = 0 To UBound($aResponse) -1 If StringInStr($aResponse[$i], "oauth_token=") Then $oauth_token = StringReplace($aResponse[$i], "oauth_token=", "") ElseIf StringInStr($aResponse[$i], "oauth_token_secret=") Then $oauth_token_secret = StringReplace($aResponse[$i], "oauth_token_secret=", "") EndIf Next $oauth_signature = $oauth_consumer_secret & "%26" & $oauth_token_secret EndFunc Func Timestamp() Local $rslt = DllCall("msvcrt.dll", "int:cdecl", "time", "int", 0) If @error = 0 Then Return $rslt[0] Return -1 EndFunc Func Nonce() Return Random(100000000, 999999999, 1) EndFunc
-
Just an update: I actually configured a client to get through the OAuth 1.0 hoops, correctly get authorized, access the xml version of the REST service and pull down the data. So, it does work and its not that complicated. The WinHTTP UDF worked great. The idea became more tedious as I tried to format the data to look like something. AFAIK, there isn't a flexible 'panel' control of sorts to stick this stuff on and let it create a scrollable view. I did use a UDF I found for scrolling which worked well but seemed like it was starting to get complicated really fast when you wanted to be able to also resize the entire gui and still make everything work. Then, the messages retrieved are of different lengths, so creating labels or something like that to display them at different sizes also became a pain. Bottom line, creating a flexible, easy-to-use gui for this sort of thing seemed to be the cumbersome part to me. I was spending way too much time on trying to get it to look decent when it was relatively simple to get a working model up and running. If anyone has some pointers on these gui issues that I mentioned or ideas, then maybe I can give it a go. Thanks for the input up till now.
-
Thanks ProgAndy! I must not have been using the right word combinations as I hadn't come across your UDF, but it looks like it would work great. A simple function could be written utilizing your WinHTTP functions so that it would be easy to do the repetitive actions of GETting and POSTing responses over and over again for the different areas of the app. Now let's see if I get interested enough to continue. ;-)
-
This is more of request for advice from the more experienced AutoIt'ers .. There is a REST service available (yammer.com) that is a great system but the Adobe AIR client is crap. No one has made a native Windows client that I can find. I don't want to spend a lot of time on it, but am thinking of playing with making one that is very basic. Searching through the forums, I see a number of functions for AutoIt to retrieve data from a web address, but little on how to POST to the service. (I did find one post but can't locate it again, although some simple tests weren't working for me with their example using wininet dll). Is it feasible to make a client app that could GET and POST data back and forth without too much trouble? The responses will come back as JSON, and I did find that someone created a UDF for working with that, so I'm assuming that wouldn't be too difficult, unless anyone has experience to say otherwise. Input is greatly appreciated. If it turned out decent enough and stable, I would release it for all to use, since its a shame there is no native option for this service.
-
Hi again, just posting the result and what I ended up doing for this and it works great! Thanks to all you who responded. This is getting called a number of times and in different ways, so I wrapped them up in separate functions to just call them out and supply the desired options. ; example insertion into the text $text = InsertTags($text, $regex, 0, 2) Func InsertTags($text, $regexp, $bef_aft = 0, $return = 1) $text = StringReplace($text, '"', '""') Switch $return Case 1 ; normal, return position 1 from regex $text = Execute('"' & StringRegExpReplace($text, $regexp, '" & MarkLocator("$1", $bef_aft) & "') & '"') Case 2 ; return positions 1 & 5 from regex $text = Execute('"' & StringRegExpReplace($text, $regexp, '" & MarkLocator("$1$7", $bef_aft) & "') & '"') Case 3 ; return positions 1 & 3 from regex $text = Execute('"' & StringRegExpReplace($text, $regexp, '" & MarkLocator("$1$3", $bef_aft) & "') & '"') EndSwitch $text = StringReplace($text, '""', '"') Return($text) EndFunc Func MarkLocator($s, $loc = 0, $get = 0) Global Static $n = 0 If $get = 0 Then $n += 1 If $loc = 1 Then Return($s & "<!--X" & $n & "-->") Else Return("<!--X" & $n & "-->" & $s) EndIf Else Return $n EndIf EndFunc
-
That works like a charm jchd. Since I will have a lot of these to run separately, I will try to wrap this into a little function to just call it for each set of matches i need to run and locators to place. It should be nice and clean. I'll post it later. JohnQ, I ran into the same thing and forgot that in this autoit function the backreference is $1 instead of 1. I may have to go that route for part of this but want to avoid it if possible so that I don't overlook any matches where perhaps there will be two markers at the same location for when it was matched for two different reasons.
-
Hello all, haven't done any AutoIt scripting in a while but can't find this particular question anywhere.. I have text files that the script will open and parse, looking with regexes for certain keywords. No problem. I need to create replacements that are unique since this will allow the script to go back and re-parse the document and easily find specific instances of each of many keywords. Basically I want to add some unique text combinations that will be "markers" inside the text to find a specific location. These can later be removed with another regex and the text stays in tact. So.. Is there a way to add a unique qualifier to the replacement text for each one using StringRegExpReplace? Even a simple sequential number added would suffice. Or, I could use StringRegExp and get an array of matches and loop through them, but how can I go to the specific match in the body of original text and perform the replacement when there can be many matches that are identical and I need each replacement to be unique? <match>text text text text text text <match> text text text text text text text <match that is different> text text text text <match still different> text text text text <match>text text. This is what I'm after: <match><MARKER1>text text text text text text <match><MARKER2> text text text text text text text <match that is different><MARKER3> text text text text <match still different><MARKER4> text text text text <match><MARKER5>text text. Thanks in advance.
-
Thanks for the input. I have checked the variable over and over and am certain it is correct. In verifying this, I think I have an idea of what it is although not certain specifically. This behavior only happens when I have only a few lines earlier opened (and closed) the file with the same name and read the contents. When doing this, then the overwrite errors. If I alternatively open and read one document and then overwrite a different doc, it works. So, is there some sort of time constraint or something that needs to be done after reading a file so that it will be able to overwrite? -Aaron
-
Hello all. I have the following lines in a script to save data to a text file. If the file does not exist, the file is created successfully. Also, if the file exists, another function using the same FileOpen but with "0" mode for read only successfully retrieves the data from the file. However, if the file already exists, and the overwrite mode is specified, it errors out. In desperation, I also tried using FileDelete first to remove it and then create it again and this also receives an error. $file_msg = FileOpen($bfile, 2) If $file_msg = -1 Then MsgBox(16, "Error 106", "We could not save the file to this location.") Return 0 Else $write = FileWrite($file_msg, $data_to_write) If $write = 0 Then MsgBox(16, "Error 107", "We could not save the data to the file selected.") EndIf ; close the file FileClose($file_msg) EndIf I have also tried using the WinAPI_CreateFile, etc and got the same error. Also moved the file being overwritten to the shared docs on the computer and it still wouldn't overwrite. Im on XP SP3 and cannot for the life of me figure this one out! Again, keep in mind that when the same code is run and the file doesn't exist yet, it is created with the data specified above. Thanks! Aaron
-
retrieve current page number in word and reader
trailwalker replied to trailwalker's topic in AutoIt General Help and Support
Nevermind on the question of how to find out the name of the controls, I just found the Window Info Tool. Great tool! So, only question remaining is, based upon the mess I will no doubt have for the various version changes in Reader, not to mention the need for constant updating as they continue to do so, is there a better way to do what I am trying to do? Thanks! Aaron -
retrieve current page number in word and reader
trailwalker replied to trailwalker's topic in AutoIt General Help and Support
Hello all and thanks again for all the help! Here is basically what I have: If $ext = ".doc" Then ; its Word doc, get page using Word UDF $oWordApp = _WordAttach($file, "FileName") $pg = $oWordApp.Application.Selection.Information($wdActiveEndPageNumber) If Not @error Then $oDoc = _WordDocGetCollection($oWordApp, 0) $page = $pg Else ; error getting page automatically $do_page = 1 EndIf ElseIf $ext = ".pdf" Then ; its a pdf, get page with page control $ViewerCtl = "[CLASSNN:Edit5]" $ViewerHd = WinWait($file) $pg = ControlGetText($ViewerHd, "", $ViewerCtl) If $pg >= 1 Then $page = $pg Else ; error getting page automatically $do_page = 1 EndIf EndIf This works beautifully with one exception. As was mentioned previously, the portion pertaining to the pdf breaks (well, gives the proper error) when its another version. So, is there any way to find out how to set that ControlGetText for various different versions of Adobe? I am not sure where to find this info. Then I can plug in a little function which will check their version first when the file is selected and set the info appropriately to get the page number. Or, if anyone has a better solution, suggestions are welcome. Thanks! -- Aaron -
retrieve current page number in word and reader
trailwalker replied to trailwalker's topic in AutoIt General Help and Support
Great! Thanks to both of you for the tips. Funny, I did quite a bit of searching on this in the forums but perhaps didn't search for it the right way as I was convinced from what I read it wouldn't be possible. I will have to look into the versions in use by all the employees that will use this. I have been sidetracked for a couple of days and will try to plug these ideas in soon and post the results. -- Aaron -
hello all. i am not so new to programming in general but new to AutoIt. I am very impressed and glad I found it. I have already created a very useful program for a friend of mine to facilitate copying and pasting info, as well as running all sorts of functions on the copied data and then grabbing the array and filling it into a proprietary software app his business uses. The one great wall I have come up against is figuring out the current page (in either Adobe Reader or Word) that the user is on when a selection is made. I have scoured the forum and found lots of info to get this far and don't see anything referring specifically to a function that could handle this. So, is there any way to obtain this information (not total pages, but current page from the cursor selection) from Adobe Reader and Word? My guess is no on both. In that case, is the only other option using OCR to grab the info from the screen (and make sure the window hasn't moved)? Also, I have seen some references to something written by someone to interact with another pdf reader, which is an option if that is possible to obtain the info that way, but I would still need it to work from Word too. Your input is greatly appreciated. Thanks, Aaron