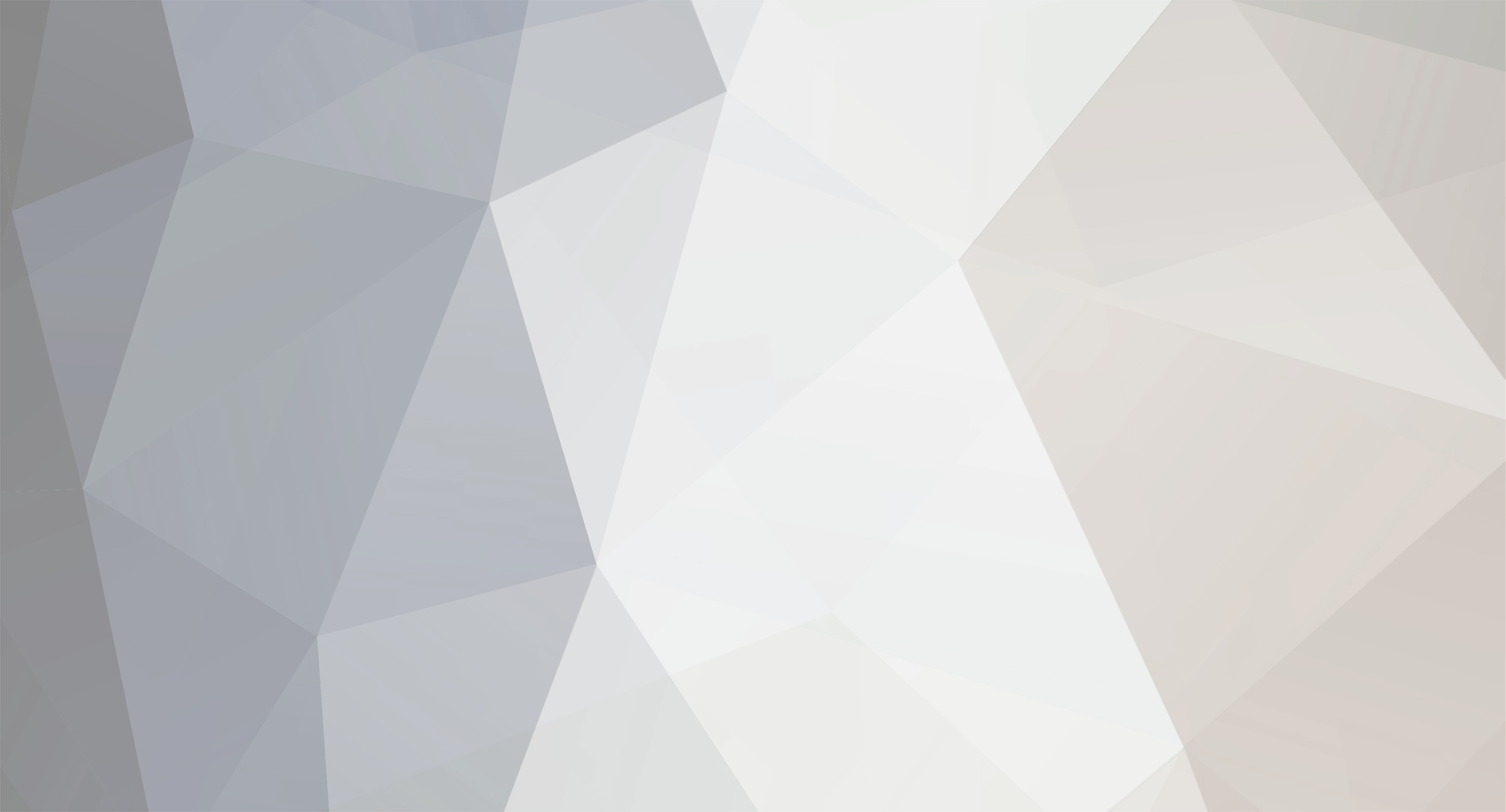
b8bboi
Active Members-
Posts
38 -
Joined
-
Last visited
Everything posted by b8bboi
-
This is great. Thanks for sharing.
-
Recursive function work around
b8bboi replied to evilertoaster's topic in AutoIt General Help and Support
I'm not exactly following you. You're trying to make a tool that works exactly like the Fill tool in Photoshop right? Then all pixels must be "connected" to each other either directly or through other pixels of the same shade. The primary logic is still the same as your recursive function. The only difference is you use an array to hold the coordinates of the pixels instead of local variables within a recursive function. From a programming stand point, every recursive function can be simulated using a stack. (That's how they're actually implemented in the first place.) -
Recursive function work around
b8bboi replied to evilertoaster's topic in AutoIt General Help and Support
This is not fast but it'll work. You can use an array. Start with one pixel. Then keep adding adjacent pixels to the array provided that they are not already in the array itself (this checking part makes it slow). Do it until you can't add adjacent pixels any more due to all of them being already in the array. To make it faster, you can use a 2 dimensional array the size of the screen to mark whether a pixel has already been added or not. -
Just tried the second one. Worked fine for me.
-
Need help with creating an On-screen Keyboard
b8bboi replied to b8bboi's topic in AutoIt GUI Help and Support
Here's a sample. #include <GUIConstants.au3> $WS_EX_NOACTIVATE = 0x08000000 Opt("GUIOnEventMode", 1) ; Change to OnEvent mode $x = GUICreate("Test OSK", 100, 100, 100, 100, $WS_POPUP, BITOR($WS_EX_NOACTIVATE, $WS_EX_TOPMOST)) $Button_2 = GUICtrlCreateButton ( "Button Test", 0, -1) GUICtrlSetOnEvent ($Button_2, "OSButtonPressed") GUISetState(@SW_SHOWNOACTIVATE) WinSetOnTop($x, "", 1) While 1 $msg = GUIGetMsg() Select Case $msg = $GUI_EVENT_CLOSE ExitLoop EndSelect Wend Func OSButtonPressed() Send("A") EndFunc -
Need help with creating an On-screen Keyboard
b8bboi replied to b8bboi's topic in AutoIt GUI Help and Support
I've found the solution. $WS_EX_NOACTIVATE. -
In a ListView, AutoIt seems to only display icons at 16x16 max, no matter what the size of the actual icon is. I've attached a screenshot to illustrate. (The actual size is 32x32). Is there a way to make it bigger? Thanks.
-
get the size of a string in pixels?
b8bboi replied to blindwig's topic in AutoIt GUI Help and Support
The problem might be with the font weight. Try explicitly setting it to 600 in both _GetTextLabelWidth and GuiCtrlSetFont. -
get the size of a string in pixels?
b8bboi replied to blindwig's topic in AutoIt GUI Help and Support
I wrote this UDF myself. It might help. Func _GetTextLabelWidth($s_WinText, $s_TextFont, $i_FontSize, $i_FontWeight = -1) Local Const $DEFAULT_CHARSET = 0 ; ANSI character set Local Const $OUT_CHARACTER_PRECIS = 2 Local Const $CLIP_DEFAULT_PRECIS = 0 Local Const $PROOF_QUALITY = 2 Local Const $FIXED_PITCH = 1 Local Const $RGN_XOR = 3 Local Const $LOGPIXELSY = 90 $h_WinTitle = "Get Label Width" If $i_FontWeight = "" Or $i_FontWeight = -1 Then $i_FontWeight = 600 ; default Font weight Local $h_GUI = GUICreate($h_WinTitle, 10, 10, -100, -100, $WS_POPUPWINDOW, $WS_EX_TOOLWINDOW) Local $hDC = DllCall("user32.dll", "int", "GetDC", "hwnd", $h_GUI) Local $intDeviceCap = DllCall("gdi32.dll", "long", "GetDeviceCaps", "int", $hDC[0], "long", $LOGPIXELSY) $intDeviceCap = $intDeviceCap[0] Local $intFontHeight = DllCall("kernel32.dll", "long", "MulDiv", "long", $i_FontSize, "long", $intDeviceCap, "long", 72) $intFontHeight = -$intFontHeight[0] Local $hMyFont = DllCall("gdi32.dll", "hwnd", "CreateFont", "int", $intFontHeight, _ "int", 0, "int", 0, "int", 0, "int", $i_FontWeight, "int", 0, _ "int", 0, "int", 0, "int", $DEFAULT_CHARSET, _ "int", $OUT_CHARACTER_PRECIS, "int", $CLIP_DEFAULT_PRECIS, _ "int", $PROOF_QUALITY, "int", $FIXED_PITCH, "str", $s_TextFont) DllCall("gdi32.dll", "hwnd", "SelectObject", "int", $hDC[0], "hwnd", $hMyFont[0]) Local $res = DllStructCreate("int;int") Local $ret = DllCall("gdi32.dll", "int", "GetTextExtentPoint32", "int", $hDC[0], "str", $s_WinText, "long", StringLen($s_WinText), "ptr", DllStructGetPtr($res)) Local $intLabelWidth = DllStructGetData($res,1) GUIDelete($h_GUI) Return $intLabelWidth EndFunc -
Need help with creating an On-screen Keyboard
b8bboi replied to b8bboi's topic in AutoIt GUI Help and Support
Zedna, thanks for the tips but GetFocus/SetFocus, et al won't work because by the time my OSK button is clicked, the focus already shifts over to Autoit. So, if I do a GetFocus now, it would just return a handle to my Autoit GUI. I hope you can see the dilemma. -
As the title said, I'm trying to create an on-screen keyboard for my touchscreen software. The easy part is to catch button click events and send keys to other apps. The hard part is how I can prevent the autoit buttons from grabbing the cursor focus away from the other app when they're clicked on. If you open Windows' osk.exe, you'll see exactly what I mean. Thanks for any input.
-
You can hide the "spotlight" and do a mouse click on the area underneath. Try this. Double-click doesn't work. You'll have to modify the code to make it work. #include <GUIConstants.au3> Opt("GUICoordMode",1) Dim $x Dim $y Dim $WinInfo Dim $MouseInfo Global $size = 200 $MouseInfo = MouseGetPos() Global $Form1 = GUICreate("AForm1", $size, $size, $MouseInfo[0]-59, $MouseInfo[1]-50, $WS_POPUP, 0) Global $label = GUICtrlCreateLabel("", 0, 0, $size, $size) WinSetTrans($Form1,"",150) GUISetState() GuiRound($Form1,0,0,$size,$size);<----- thanks gaFrost While 1 $msg = GUIGetMsg(1) Select Case $msg[0] = $GUI_EVENT_MOUSEMOVE $WinInfo = WinGetPos($Form1) $MouseInfo = MouseGetPos() $x = ($WinInfo[2]/2) $y = ($WinInfo[3]/2) WinMove($Form1,"",$MouseInfo[0]-$x+1,$MouseInfo[1]-$y+1) Case $msg[0] = $label MouseClicked() Case $msg[0] = $GUI_EVENT_CLOSE Exit EndSelect WEnd Func MouseClicked() Local $mousepos = MouseGetPos() GUISetState(@SW_HIDE, $Form1) Sleep(100) MouseClick("", $mousepos[0], $mousepos[1], 1, 1) GUISetState(@SW_SHOW, $Form1) EndFunc ;=============================================================================== ; ; Function Name: GuiRoundHole() ; Description: Rounds the corners of a window ; Parameter(s): $h_win ; $i_x1 ; $i_y1 ; $i_x3 ; $i_y3 ; Requirement(s): AutoIt3 ; Return Value(s): ; Author(s): gaFrost ; ;=============================================================================== Func GuiRound($h_win, $i_x1, $i_y1, $i_x3, $i_y3) Local $halfx = Int(($i_x3 - $i_x1) / 2) Local $halfy = Int(($i_y3 - $i_y1) / 2) Local $holesize = 3 Local $outer_rgn = DllCall("gdi32.dll", "long", "CreateEllipticRgn", "long", $i_x1, "long", $i_y1, "long", $i_x3 , "long", $i_y3) If $outer_rgn[0] Then Local $ret3 = DllCall("user32.dll", "long", "SetWindowRgn", "hwnd", $h_win, "long", $outer_rgn[0], "int", 1) If $ret3[0] Then Return 1 Else Return 0 EndIf Else Return 0 EndIf EndFunc ;==>_GuiRoundHole
-
Here you go. Just change the size, location, etc. to your liking. Also, add some error checking just in case. #include <GUIConstants.au3> Opt("GUICoordMode",1) Dim $x Dim $y Dim $WinInfo Dim $MouseInfo Global $size = 200 $MouseInfo = MouseGetPos() $Form1 = GUICreate("AForm1", $size, $size, $MouseInfo[0]-59, $MouseInfo[1]-50, $WS_POPUP, 0) WinSetTrans($Form1,"",150) GUISetState() GuiRoundHole($Form1,0,0,$size,$size);<----- thanks gaFrost While 1 $msg = GUIGetMsg(1) Select Case $msg[0] = $GUI_EVENT_MOUSEMOVE $WinInfo = WinGetPos($Form1) $MouseInfo = MouseGetPos() $x = ($WinInfo[2]/2) $y = ($WinInfo[3]/2) WinMove($Form1,"",$MouseInfo[0]-$x+1,$MouseInfo[1]-$y+1) Case $msg[0] = $GUI_EVENT_CLOSE Exit EndSelect WEnd ;=============================================================================== ; ; Function Name: GuiRoundHole() ; Description: Rounds the corners of a window ; Parameter(s): $h_win ; $i_x1 ; $i_y1 ; $i_x3 ; $i_y3 ; Requirement(s): AutoIt3 ; Return Value(s): ; Author(s): gaFrost ; ;=============================================================================== Func GuiRoundHole($h_win, $i_x1, $i_y1, $i_x3, $i_y3) Local $halfx = Int(($i_x3 - $i_x1) / 2) Local $halfy = Int(($i_y3 - $i_y1) / 2) Local $holesize = 3 Local $outer_rgn = DllCall("gdi32.dll", "long", "CreateEllipticRgn", "long", $i_x1, "long", $i_y1, "long", $i_x3 , "long", $i_y3) Local $inner_rgn = DllCall("gdi32.dll", "long", "CreateEllipticRgn", "long", $i_x1+$halfx-$holesize, "long", $i_y1+$halfy-$holesize, "long", $i_x1+$halfx+$holesize, "long", $i_y1+$halfy+$holesize) If $outer_rgn[0] and $inner_rgn[0] Then Local $combined_rgn = DllCall("gdi32.dll", "long", "CreateEllipticRgn", "long", 0, "long", 0, "long", 0, "long", 0) DllCall("gdi32.dll", "long", "CombineRgn", "long", $combined_rgn[0], "long", $outer_rgn[0], "long", $inner_rgn[0], "int", 4) Local $ret3 = DllCall("user32.dll", "long", "SetWindowRgn", "hwnd", $h_win, "long", $combined_rgn[0], "int", 1) If $ret3[0] Then Return 1 Else Return 0 EndIf Else Return 0 EndIf EndFunc ;==>_GuiRoundHole
-
get inputs from another program
b8bboi replied to dark_jedi's topic in AutoIt General Help and Support
StdoutRead might work. -
How to embed another window into my GUI
b8bboi replied to BigDaddyO's topic in AutoIt GUI Help and Support
Not sure if this is what you're looking for but you can set the remote desktop GUI as a child of one of your GUIs and do whatever you want with it. -
Concatenate Variable Names?
b8bboi replied to MasterRaistlin's topic in AutoIt General Help and Support
GuiCtrlSetData takes a control handle, not just a name. You can try something like this. $iIndex = 6 For $x = 1 to $iIndex $sTempSymbolInput = $SymbolInput & $x ; Wanting end result of $sTempSymbolInput to be $SymbolInput1 $temp_ctrl = ControlGetHandle("Your Window Name", "", $sTempSymbolinput) GuiCtrlSetData($temp_ctrl, $symArray[$x - 1]) Next -
Yeah, using background image and invisible buttons is nice but how do we create the "button pushed" effect? Most skinnable GUIs let you define an "On" image (that's when the button is "pushed") and an "Off" image (which is the background that we already have). How do we achieve the effect of having an "On" image? If there was a function that would load a portion of an image (from the On image) right on top of the background, that would be perfect. However, I'm not aware of anything like that.
-
Ben, a couple of questions. 1. Can a coroutine access global variables in the parent thread or do you have to use _CoSend()? 2. If the parent process is killed forcefully, ie. via Task Manager, is the coroutine process killed as well?
-
so, following Valik's advice, I've tried the following but it didn't work. The GetTextExtentPoint32 function always returns a false value and doesn't return the actual size fo the label. #include <GUIConstants.au3> $myGui = GUICreate("Test Label Size") $WM_GETFONT = 0x31 $text = "ABCDEFGHIJKLMN" $hText = GUICtrlCreateLabel ($text, 10, 10, 100, 80, $SS_SIMPLE) GUICtrlSetFont(-1, 20, 600, "", "Arial Narrow") GUISetState () $MyHDC = DLLCall("user32.dll","int","GetDC","hwnd",$hText) $hFont = DllCall("user32.dll", "ptr", "SendMessage", "hwnd", $hText, "int", $WM_GETFONT, "int", 0, "int", 0) $hFont = $hFont[1] $hOld = DllCall("gdi32.dll", "Hwnd", "SelectObject", "int", $MyHDC[0], "ptr", $hFont) $res = DllStructCreate("long;long") $ret = DllCall("gdi32.dll", "int", "GetTextExtentPoint32", "int", $MyHDC[0], "str", $text, "long", StringLen($text), "ptr", DllStructGetPtr($res)) MsgBox(0, "Get Size", "Return Status="&$ret[0]&" | width="&DllStructGetData($res,0)) DLLCall("user32.dll","int","ReleaseDC","int",$MyHDC[0],"hwnd",$hText) Could anyone see why? Thanks.
-
I'm using GuiCtrCreateLabel to try to simulate a text input control. The reason why I don't want to use a simple input control is that I want to have a transparent background. $GUI_BKCOLOR_TRANSPARENT doesn't work on Input boxes. The label control is gonna have a fixed width. It also gets updated when user types into another (hidden) input box. The problem is I need to know when the text label is clipped so that I can trim the first few characters from the string. This way the right most characters are always visible. I hope I'm clear enough. Appreciate any help.
-
Is There A Way To Create A "hole" In Your Gui Window?
b8bboi replied to b8bboi's topic in AutoIt GUI Help and Support
That's awesome. I'll play around with your code to see if I can cut a rectangle, not a circle like in the example. Thanks. Edit: figured it out. Thanks again. -
Is There A Way To Create A "hole" In Your Gui Window?
b8bboi replied to b8bboi's topic in AutoIt GUI Help and Support
Yeah, that's EXACTLY what I said. I'm looking for a more elegant solution. Say, if you want to create a background image for your window, you won't have to chop it up, too. -
As the topic title said, is there a way to create a square "hole" in the GUI window so that everything behind the window will show through, even mouse clicks? Right now, my temporary solution is to create a hidden parent window and a 4 child windows positioned around the "hole." Of course, that's not elegant at all. Any help is appreiciated.
-
Gif Transparency And Winsettrans Won't Work Together
b8bboi replied to b8bboi's topic in AutoIt GUI Help and Support
gafrost, I tried that and it didn't work. I still get the white background. I guess this is just a limitation of WinSetTrans.